Table of Contents
In the world of computer programming, different paradigms have emerged to solve complex problems efficiently. One such paradigm that has gained significant popularity and recognition is “Functional Programming.” Unlike the more traditional “imperative programming,” functional programming focuses on the evaluation of functions to perform tasks and emphasizes the use of pure functions, immutability, and higher-order functions. This article delves into the fundamentals of functional programming and its benefits in software development.
What is Functional Programming?
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. In other words, it emphasizes writing code in a declarative style where you define what you want to achieve rather than specifying how to achieve it.
The central concept in functional programming is the use of “pure functions.” A pure function is a function that always produces the same output for the same input and has no side effects. This means that pure functions do not modify external variables, perform input/output operations, or rely on mutable data. Given the same input, a pure function will consistently return the same output, making it easy to reason about and test.
Key Concepts in Functional Programming:
- Immutability: In functional programming, data is immutable, meaning once it is created, it cannot be changed. Instead of modifying existing data structures, functional programs create new ones with the desired changes, ensuring the integrity of the original data.
- Higher-Order Functions: Functional programming encourages the use of higher-order functions, which are functions that can take other functions as arguments or return them as results. This enables a higher level of abstraction and code reusability.
- Recursion: Rather than using loops, functional programming often employs recursion to achieve repetition and iteration. Recursive functions call themselves with modified parameters until a base condition is met.
- Referential Transparency: This property implies that a function’s output can be replaced with its computed value without affecting the program’s behavior. In other words, a function’s behavior depends solely on its inputs and not on any external state.
- Lazy Evaluation: Functional programming languages often support lazy evaluation, where expressions are not evaluated until their results are needed. This can lead to more efficient programs, especially when working with infinite data structures.
Benefits of Functional Programming:
- Concurrency and Parallelism: Functional programming’s emphasis on immutability and pure functions reduces the chances of race conditions and makes it easier to reason about concurrent code. As a result, functional programs can be more straightforward to parallelize, taking advantage of multi-core processors.
- Testability and Debugging: Pure functions, due to their lack of side effects, are easier to test as they produce consistent results for a given input. Also, functional programming encourages breaking down complex problems into smaller, composable functions, facilitating unit testing.
- Readability and Maintainability: Declarative code in functional programming is often more readable and expressive. The focus on immutability and the absence of explicit loops can lead to cleaner and more maintainable code.
- Modularity and Reusability: Higher-order functions and the emphasis on composing small functions into larger ones promote code reuse and modular design. This makes it easier to refactor and extend functionality.
- No Shared State Bugs: Since functional programming avoids mutable data and shared state, many common bugs related to changing state are eliminated.
Popular Functional Programming Languages:
Several programming languages support functional programming to varying degrees. Some of the most well-known functional programming languages include:
- Haskell: Haskell is a purely functional language known for its strong type system and mathematical approach to programming.
- Clojure: Clojure is a modern Lisp dialect that runs on the Java Virtual Machine (JVM) and embraces immutability and functional programming concepts.
- Erlang: Erlang is designed for concurrent, fault-tolerant systems and is widely used in telecommunications and distributed systems.
- Scala: Scala is a hybrid functional and object-oriented language that runs on the JVM and is fully interoperable with Java.
- Elixir: Elixir is built on top of Erlang’s virtual machine and is known for its scalability and fault tolerance.
Conclusion:
Functional programming offers a fresh perspective on developing software by focusing on the purity of functions, immutability, and the absence of side effects. By adhering to these principles, functional programming languages can produce robust, testable, and maintainable code that scales well in concurrent and parallel environments. While functional programming might not be the best fit for all types of projects, understanding its concepts can broaden a programmer’s horizons and lead to more elegant and efficient solutions in many domains. As the software development landscape evolves, functional programming will undoubtedly continue to play a crucial role in shaping the future of programming paradigms.
What is functional programming?
Functional programming (FP) is a programming paradigm for developing software using functions.Functional programming languages are specially designed to handle symbolic computation and list processing applications. Functional programming is based on mathematical functions.
What are the Characteristics of Functional Programming ?
Characteristics of Functional Programming are as follows
1.Functional programming method focuses on results, not the process
2.Emphasis is on what is to be computed
3.Data is immutable
4.Functional programming Decompose the problem into ‘functions
5.It is built on the concept of mathematical functions which uses conditional expressions and recursion to do 6.perform the calculation
7.It does not support iteration like loop statements and conditional statements like If-Else.
What are the benefits of functional programming
1.Allows you to avoid confusing problems and errors in the code
2.Easier to test and execute Unit testing and debug FP Code.
3.Parallel processing and concurrency
4.Hot code deployment and fault tolerance
5.Offers better modularity with a shorter code
6.Increased productivity of the developer
7.Supports Nested Functions
8.Functional Constructs like Lazy Map & Lists, etc.
9.Allows effective use of Lambda Calculus
What are the Limitations of Functional Programming
1.Functional programming paradigm is not easy, so it is difficult to understand for the beginner
2.Hard to maintain as many objects evolve during the coding
3.Needs lots of mocking and extensive environmental setup
4.Re-use is very complicated and needs constantly refactoring
5.Objects may not represent the problem correctly
Terminology and Concepts related to Functional Programming
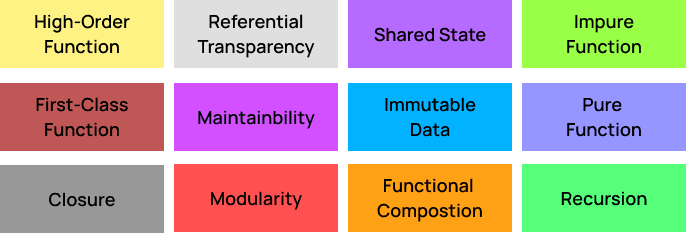
1.Immutable Data
Immutable Data means that you should easily able to create data structures instead of modifying ones which is already exist.
2.Referential transparency
Functional programs should perform operations just like as if it is for the first time. So, you will know what may or may not have happened during the program’s execution, and its side effects. In FP term it is called Referential transparency.
3.Modularity
Modular design increases productivity. Small modules can be coded quickly and have a greater chance of re-use which surely leads to faster development of programs. Apart from it, the modules can be tested separately which helps you to reduce the time spent on unit testing and debugging.
4.Maintainability
Maintainability is a simple term which means FP programming is easier to maintain as you don’t need to worry about accidentally changing anything outside the given function.
5.First-class function
‘First-class function’ is a definition, attributed to programming language entities that have no restriction on their use. Therefore, first-class functions can appear anywhere in the program.
6.Closure
The closure is an inner function which can access variables of parent function’s, even after the parent function has executed.
7.Higher-order functions
Higher-order functions either take other functions as arguments or return them as results.
Higher-order functions allow partial applications or currying. This technique applies a function to its arguments one at a time, as each application returning a new function which accepts the next argument.
8.Pure function
A ‘Pure function’ is a function whose inputs are declared as inputs and none of them should be hidden. The outputs are also declared as outputs.
Pure functions act on their parameters. It is not efficient if not returning anything. Moreover, it offers the same output for the given parameters
Example:
Function Pure(a,b) { return a+b; }
9.Impure functions
Impure functions exactly in the opposite of pure. They have hidden inputs or output; it is called impure. Impure functions cannot be used or tested in isolation as they have dependencies.
Example
int z; function notPure(){ z = z+10; }
10.Function Composition
Function composition is combining 2 or more functions to make a new one.
11.Shared States
Shared states is an importance concept in OOP programming. Basically, It’s adding properties to objects. For example, if a HardDisk is an Object, Storage Capacity and Disk Size can be added as properties.
Side Effects
Side effects are any state changes that occur outside of a called function. The biggest goal of any FP programming language is to minimize side effects, by separating them from the rest of the software code. In FP programming It is vital to take away side effects from the rest of your programming logic.
12.Recursion
In the functional programming paradigm, there is no for and while loops. Instead, functional programming languages rely on recursion for iteration. Recursion is implemented using recursive functions, which repetitively call themselves until the base case is reached.
What are Functional Programming Languages
The objective of any FP language is to mimic the mathematical functions. However, the basic process of computation is different in functional programming.
Here, are some most prominent Functional programming languages:
1.Scala
2.Erlang
3.SQL
4.Haskell
5..SML
6.Clojure
7.Mathematica
8.Clean
9.F#
10.ML/OCaml Lisp / Scheme
11.XSLT
Functional Programming vs. Object-oriented Programming
Functional Programming | OOP |
---|---|
FP uses Immutable data. | OOP uses Mutable data. |
Follows Declarative Programming based Model. | Follows Imperative Programming Model. |
What it focuses is on: “What you are doing. in the programme.” | What it focuses is on “How you are doing your programming.” |
Supports Parallel Programming. | No supports for Parallel Programming. |
Its functions have no-side effects. | Method can produce many side effects. |
Flow Control is performed using function calls & function calls with recursion. | Flow control process is conducted using loops and conditional statements. |
Execution order of statements is not very important. | Execution order of statements is important. |
Supports both “Abstraction over Data” and “Abstraction over Behavior.” | Supports only “Abstraction over Data”. |
Let’s understand the functional programming with a video by Hitesh Choudhary Sir!
Hope you this article on what is functional programming is useful to you .Don’t forget to share with your friends. Check out our tutorials on