Table of Contents
In this tutorial, you’ll learn how to Create Servlet Application using tomcat server in step-wise manner.
To create a Servlet application you need to follow the below-mentioned steps. These steps are common for all the Web servers. In this example, we are using Apache Tomcat server. Apache Tomcat is an open-source web server for testing servlets and JSP technology.
Steps to Create Servlet Application using tomcat server
After installing Tomcat Server on your machine follow the below mentioned steps :
- Create directory structure for your application.
- Create a Servlet
- Compile the Servlet
- Create Deployement Descriptor for your application
- Start the server and deploy the application
All these 5 steps are explained in details below, lets create our first Servlet Application.
Step 1: Creating the Directory Structure
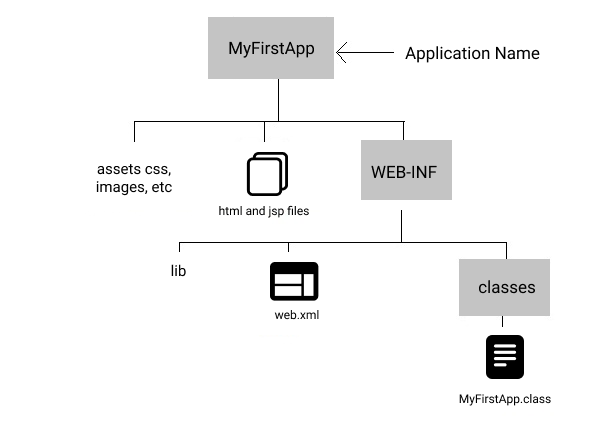
- Create the above directory structure inside Apache-Tomcat\webapps directory. All HTML, static files(images, css etc) are kept directly under Web application folder. While all the Servlet classes are kept insideÂ
classes
 folder.
- TheÂ
web.xml
 (deployement descriptor) file is kept underÂWEB-INF
 folder.
Step2 : Creating a Servlet
There are three different ways to create a servlet.
- By implementing Servlet interface
- By extending GenericServlet class
- By extending HttpServlet class
But mostly a servlet is created by extending HttpServlet abstract class. As discussed earlier HttpServlet gives the definition of service()
 method of the Servlet interface. The servlet class that we will create should not override service()
 method. Our servlet class will override only doGet()
 or doPost()
 method.
When a request comes in for the servlet, the Web Container calls the servlet’s service()
 method and depending on the type of request the service()
 method calls either the doGet()
 or doPost()
 method.
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.*;
public class HelloWorld extends HttpServlet
{
public void doGet(HttpServletRequest req,HttpServletResponse resp)
throws IOException, ServletException {
resp.setContentType("text/html");
PrintWriter out = resp.getWriter();
out.println("<html><body>")
out.println("<h1>Hello and welcome to HttpServlet</h1>");
out.println("</body></html>");
}
}
Compile the java file to get a class file.
Step 3 : Compiling a Servlet
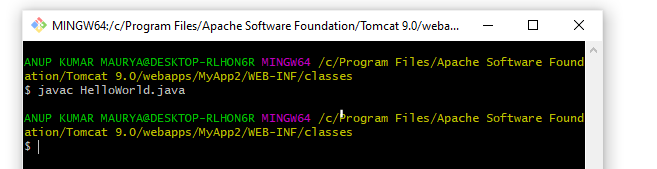
Step 4 : Create Deployment Descriptor
Deployment Descriptor(DD)Â is an XML document that is used by Web Container to run Servlets and JSP pages. DD is used for several important purposes such as:
- Mapping URL to Servlet class.
- Initializing parameters.
- Defining Error page.
- Security roles.
- Declaring tag libraries.
We will discuss all these in detail later. Now we will see how to create a simple web.xml file for our web application.
<web-app>
<servlet>
<servlet-name>HelloWorld</servlet-name>
<servlet-class>HelloWorld</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>HelloWorld</servlet-name>
<url-pattern>/welcome</url-pattern>
</servlet-mapping>
</web-app>
Now Let’s discuss about all the tag used above in web.xml file
<web-app>Â represents the whole application. |
<servlet>Â is sub element of <web-app> and represents the servlet. |
<servlet-name>Â is sub element of <servlet> represents the name of the servlet. |
<servlet-class>Â is sub element of <servlet> represents the class of the servlet. |
<servlet-mapping>Â is sub element of <web-app>. It is used to map the servlet. |
<url-pattern>Â is sub element of <servlet-mapping>. This pattern is used at client side to invoke the servlet. |
Step 5: Start the server and deploy the application
To start Apache Tomcat server, double click on the startup.bat file under Tomcat 9.0\bin directory. and
Open Browser and type http:localhost:9999/MyApp2/welcome
Note: Here ,I am not using default port 8080.
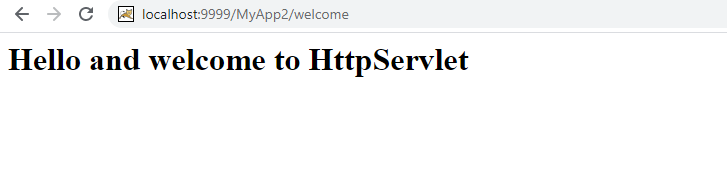
Hope you have enjoyed this tutorial ,Love to hear your honest feedback!
Happy Programming 🙂