Table of Contents
Java interview Questions . Topic wise Java Questions . Basics . OOPS, JSP ,JDBC and many more .
Java is a general-purpose, class-based, object-oriented programming language designed for having lesser implementation dependencies. It is a computing platform for application development.
Q1. Explain JDK, JRE and JVM?
JDK | JRE | JVM |
It stands for Java Development Kit. | It stands for Java Runtime Environment. | It stands for Java Virtual Machine. |
It is the tool necessary to compile, document and package Java programs. | JRE refers to a runtime environment in which Java bytecode can be executed. | It is an abstract machine. It is a specification that provides a run-time environment in which Java bytecode can be executed. |
It contains JRE + development tools. | It’s an implementation of the JVM which physically exists. | JVM follows three notations: Specification, Implementation, and Runtime Instance. |
Q2. Explain public static void main(String args[]) in Java.
main() in Java is the entry point for any Java program. It is always written as public static void main(String[] args).
- public: Public is an access modifier, which is used to specify who can access this method. Public means that this Method will be accessible by any Class.
- static: It is a keyword in java which identifies it is class-based. main() is made static in Java so that it can be accessed without creating the instance of a Class. In case, main is not made static then the compiler will throw an error as main() is called by the JVM before any objects are made and only static methods can be directly invoked via the class.
- void: It is the return type of the method. Void defines the method which will not return any value.
- main: It is the name of the method which is searched by JVM as a starting point for an application with a particular signature only. It is the method where the main execution occurs.
- String args[]: It is the parameter passed to the main method.
Q3. Why Java is platform independent?
Java is called platform independent because of its byte codes which can run on any system irrespective of its underlying operating system.
Q4. Why Java is not 100% Object-oriented?
Java is not 100% Object-oriented because it makes use of eight primitive data types such as boolean, byte, char, int, float, double, long, short which are not objects.
Q5. What are wrapper classes in Java?
Wrapper classes convert the Java primitives into the reference types (objects). Every primitive data type has a class dedicated to it. These are known as wrapper classes because they “wrap” the primitive data type into an object of that class. Refer to the below image which displays different primitive type, wrapper class and constructor argument.
Q6. What are constructors in Java?
In Java, constructor refers to a block of code which is used to initialize an object. It must have the same name as that of the class. Also, it has no return type and it is automatically called when an object is created.
There are two types of constructors:
- Default Constructor: In Java, a default constructor is the one which does not take any inputs. In other words, default constructors are the no argument constructors which will be created by default in case you no other constructor is defined by the user. Its main purpose is to initialize the instance variables with the default values. Also, it is majorly used for object creation.
- Parameterized Constructor: The parameterized constructor in Java, is the constructor which is capable of initializing the instance variables with the provided values. In other words, the constructors which take the arguments are called parameterized constructors.
Q7. What is singleton class in Java and how can we make a class singleton?
Singleton class is a class whose only one instance can be created at any given time, in one JVM. A class can be made singleton by making its constructor private.
Q8. What is the difference between Array list and vector in Java?
ArrayList | Vector |
---|---|
Array List is not synchronized. | Vector is synchronized. |
Array List is fast as it’s non-synchronized. | Vector is slow as it is thread safe. |
If an element is inserted into the Array List, it increases its Array size by 50%. | Vector defaults to doubling size of its array. |
Array List does not define the increment size. | Vector defines the increment size. |
Array List can only use Iterator for traversing an Array List. | Vector can use both Enumeration and Iterator for traversing. |
Q9. What is the difference between equals() and == in Java?
Equals() method is defined in Object class in Java and used for checking equality of two objects defined by business logic.
“==” or equality operator in Java is a binary operator provided by Java programming language and used to compare primitives and objects. public boolean equals(Object o) is the method provided by the Object class. The default implementation uses == operator to compare two objects. For example: method can be overridden like String class. equals() method is used to compare the values of two objects.
Q10. What are the differences between Heap and Stack Memory in Java?
The major difference between Heap and Stack memory are:
Features | Stack | Heap |
---|---|---|
Memory | Stack memory is used only by one thread of execution. | Heap memory is used by all the parts of the application. |
Access | Stack memory can’t be accessed by other threads. | Objects stored in the heap are globally accessible. |
Memory Management | Follows LIFO manner to free memory. | Memory management is based on the generation associated with each object. |
Lifetime | Exists until the end of execution of the thread. | Heap memory lives from the start till the end of application execution. |
Usage | Stack memory only contains local primitive and reference variables to objects in heap space. | Whenever an object is created, it’s always stored in the Heap space. |
Q11. What is a package in Java? List down various advantages of packages.
Packages in Java, are the collection of related classes and interfaces which are bundled together. By using packages, developers can easily modularize the code and optimize its reuse. Also, the code within the packages can be imported by other classes and reused. Below I have listed down a few of its advantages:
- Packages help in avoiding name clashes
- They provide easier access control on the code
- Packages can also contain hidden classes which are not visible to the outer classes and only used within the package
- Creates a proper hierarchical structure which makes it easier to locate the related classes
Q12. Why pointers are not used in Java?
Java doesn’t use pointers because they are unsafe and increases the complexity of the program. Since, Java is known for its simplicity of code, adding the concept of pointers will be contradicting. Moreover, since JVM is responsible for implicit memory allocation, thus in order to avoid direct access to memory by the user, pointers are discouraged in Java.
Q13. What is JIT compiler in Java?
JIT stands for Just-In-Time compiler in Java. It is a program that helps in converting the Java bytecode into instructions that are sent directly to the processor. By default, the JIT compiler is enabled in Java and is activated whenever a Java method is invoked. The JIT compiler then compiles the bytecode of the invoked method into native machine code, compiling it “just in time” to execute. Once the method has been compiled, the JVM summons the compiled code of that method directly rather than interpreting it. This is why it is often responsible for the performance optimization of Java applications at the run time.
Q14. What are access modifiers in Java?
In Java, access modifiers are special keywords which are used to restrict the access of a class, constructor, data member and method in another class. Java supports four types of access modifiers:
- Default
- Private
- Protected
- Public
Modifier | Default | Private | Protected | Public |
Same class | YES | YES | YES | YES |
Same Package subclass | YES | NO | YES | YES |
Same Package non-subclass | YES | NO | YES | YES |
Different package subclass | NO | NO | YES | YES |
Different package non-subclass | NO | NO | NO | YES |
Q15. Define a Java Class.
A class in Java is a blueprint which includes all your data. A class contains fields (variables) and methods to describe the behavior of an object. Let’s have a look at the syntax of a class.
class Abc {
member variables // class body methods
}
Q16. What is an object in Java and how is it created?
An object is a real-world entity that has a state and behavior. An object has three characteristics:
- State
- Behavior
- Identity
An object is created using the ‘new’ keyword. For example:
ClassName obj = new ClassName();
Q17. What is Object Oriented Programming?
Object-oriented programming or popularly known as OOPs is a programming model or approach where the programs are organized around objects rather than logic and functions. In other words, OOP mainly focuses on the objects that are required to be manipulated instead of logic. This approach is ideal for the programs large and complex codes and needs to be actively updated or maintained.
Q18. What are the main concepts of OOPs in Java?
Object-Oriented Programming or OOPs is a programming style that is associated with concepts like:
- Inheritance: Inheritance is a process where one class acquires the properties of another.
- Encapsulation: Encapsulation in Java is a mechanism of wrapping up the data and code together as a single unit.
- Abstraction: Abstraction is the methodology of hiding the implementation details from the user and only providing the functionality to the users.
- Polymorphism: Polymorphism is the ability of a variable, function or object to take multiple forms.
Q19. What is the difference between a local variable and an instance variable?
In Java, a local variable is typically used inside a method, constructor, or a block and has only local scope. Thus, this variable can be used only within the scope of a block. The best benefit of having a local variable is that other methods in the class won’t be even aware of that variable.
Example
if(x > 100)
{ String test = "Techarge";
}
Whereas, an instance variable in Java, is a variable which is bounded to its object itself. These variables are declared within a class, but outside a method. Every object of that class will create it’s own copy of the variable while using it. Thus, any changes made to the variable won’t reflect in any other instances of that class and will be bound to that particular instance only.
class Test{
public String EmpName;
public int empAge;
}
Q20. Differentiate between the constructors and methods in Java?
Methods | Constructors |
1. Used to represent the behavior of an object | 1. Used to initialize the state of an object |
2. Must have a return type | 2. Do not have any return type |
3. Needs to be invoked explicitly | 3. Is invoked implicitly |
4. No default method is provided by the compiler | 4. A default constructor is provided by the compiler if the class has none |
5. Method name may or may not be same as class name | 5. Constructor name must always be the same as the class name |
Q21. What is final keyword in Java?
final is a special keyword in Java that is used as a non-access modifier. A final variable can be used in different contexts such as:
- final variable
When the final keyword is used with a variable then its value can’t be changed once assigned. In case the no value has been assigned to the final variable then using only the class constructor a value can be assigned to it.
- final method
When a method is declared final then it can’t be overridden by the inheriting class.
- final class
When a class is declared as final in Java, it can’t be extended by any subclass class but it can extend other class.
Q22. What is the difference between break and continue statements?
break | continue |
1. Can be used in switch and loop (for, while, do while) statements | 1. Can be only used with loop statements |
2. It causes the switch or loop statements to terminate the moment it is executed | 2. It doesn’t terminate the loop but causes the loop to jump to the next iteration |
3. It terminates the innermost enclosing loop or switch immediately | 3. A continue within a loop nested with a switch will cause the next loop iteration to execute |
Example break:
for (int i = 0; i < 5; i++)
{ if (i == 3)
{break;
}
System.out.println(i);
}
Example continue:
for (int i = 0; i < 5; i++) {
if(i == 2)
{continue;
}
System.out.println(i);
}
Q23.What is an infinite loop in Java? Explain with an example.
An infinite loop is an instruction sequence in Java that loops endlessly when a functional exit isn’t met. This type of loop can be the result of a programming error or may also be a deliberate action based on the application behavior. An infinite loop will terminate automatically once the application exits.
For example:
public class InfiniteForLoopDemo
{public static void main(String[] arg)
{for(;;)
System.out.println("Welcome to Edureka!");// To terminate this program press ctrl + c in the console.
}}
Q24. What is the difference between this() and super() in Java?
In Java, super() and this(), both are special keywords that are used to call the constructor.
this() | super() |
1. this() represents the current instance of a class | 1. super() represents the current instance of a parent/base class |
2. Used to call the default constructor of the same class | 2. Used to call the default constructor of the parent/base class |
3. Used to access methods of the current class | 3. Used to access methods of the base class |
4. Used for pointing the current class instance | 4. Used for pointing the superclass instance |
5. Must be the first line of a block | 5. Must be the first line of a block |
Q26. Differentiate between static and non-static methods in Java.
Static Method | Non-Static Method |
1. The static keyword must be used before the method name | 1. No need to use the static keyword before the method name |
2. It is called using the class (className.methodName) | 2. It is can be called like any general method |
3. They can’t access any non-static instance variables or methods | 3. It can access any static method and any static variable without creating an instance of the class |
Q27. What is constructor chaining in Java?
In Java, constructor chaining is the process of calling one constructor from another with respect to the current object. Constructor chaining is possible only through legacy where a subclass constructor is responsible for invoking the superclass’ constructor first. There could be any number of classes in the constructor chain. Constructor chaining can be achieved in two ways:
- Within the same class using this()
- From base class using super()
Q28. Difference between String, StringBuilder, and StringBuffer.
Factor | String | StringBuilder | StringBuffer |
Storage Area | Constant String Pool | Heap Area | Heap Area |
Mutability | Immutable | Mutable | Mutable |
Thread Safety | Yes | No | Yes |
Performance | Fast | More efficient | Less efficient |
Q29. What is a classloader in Java?
The Java ClassLoader is a subset of JVM (Java Virtual Machine) that is responsible for loading the class files. Whenever a Java program is executed it is first loaded by the classloader. Java provides three built-in classloaders:
- Bootstrap ClassLoader
- Extension ClassLoader
- System/Application ClassLoader
Q30. Why Java Strings are immutable in nature?
In Java, string objects are immutable in nature which simply means once the String object is created its state cannot be modified. Whenever you try to update the value of that object instead of updating the values of that particular object, Java creates a new string object. Java String objects are immutable as String objects are generally cached in the String pool. Since String literals are usually shared between multiple clients, action from one client might affect the rest. It enhances security, caching, synchronization, and performance of the application.
Q31. What is the difference between an array and an array list?
Array | ArrayList |
---|---|
Cannot contain values of different data types | Can contain values of different data types. |
Size must be defined at the time of declaration | Size can be dynamically changed |
Need to specify the index in order to add data | No need to specify the index |
Arrays are not type parameterized | Arraylists are type |
Arrays can contain primitive data types as well as objects | Arraylists can contain only objects, no primitive data types are allowed |
Q32. What is a Map in Java?
In Java, Map is an interface of Util package which maps unique keys to values. The Map interface is not a subset of the main Collection interface and thus it behaves little different from the other collection types. Below are a few of the characteristics of Map interface:
- Map doesn’t contain duplicate keys.
- Each key can map at max one value.
Q33. What is collection class in Java? List down its methods and interfaces.
In Java, the collection is a framework that acts as an architecture for storing and manipulating a group of objects. Using Collections you can perform various tasks like searching, sorting, insertion, manipulation, deletion, etc. Java collection framework includes the following:
- Interfaces
- Classes
- Methods
OOPs Java Question
Q1. What is Polymorphism?
Polymorphism is briefly described as “one interface, many implementations”. Polymorphism is a characteristic of being able to assign a different meaning or usage to something in different contexts – specifically, to allow an entity such as a variable, a function, or an object to have more than one form. There are two types of polymorphism:
- Compile time polymorphism
- Run time polymorphism
Compile time polymorphism is method overloading whereas Runtime time polymorphism is done using inheritance and interface.
Q2. What is runtime polymorphism or dynamic method dispatch?
In Java, runtime polymorphism or dynamic method dispatch is a process in which a call to an overridden method is resolved at runtime rather than at compile-time. In this process, an overridden method is called through the reference variable of a superclass. Let’s take a look at the example below to understand it better.
class Car {
void run()
{
System.out.println(“car is running”);
}
}
class Audi extends Car {
void run()
{
System.out.prinltn(“Audi is running safely with 100km”);
}
public static void main(String args[])
{
Car b= new Audi(); //upcasting
b.run();
}
}
Q3. What is abstraction in Java?
Abstraction refers to the quality of dealing with ideas rather than events. It basically deals with hiding the details and showing the essential things to the user. Thus you can say that abstraction in Java is the process of hiding the implementation details from the user and revealing only the functionality to them. Abstraction can be achieved in two ways:
- Abstract Classes (0-100% of abstraction can be achieved)
- Interfaces (100% of abstraction can be achieved)
Q4. What do you mean by an interface in Java?
An interface in Java is a blueprint of a class or you can say it is a collection of abstract methods and static constants. In an interface, each method is public and abstract but it does not contain any constructor. Thus, interface basically is a group of related methods with empty bodies. Example:
public interface Animal {
public void eat();
public void sleep();
public void run();
}
Q5. What is the difference between abstract classes and interfaces?
Abstract Class | Interfaces |
---|---|
An abstract class can provide complete, default code and/or just the details that have to be overridden | An interface cannot provide any code at all, just the signature |
In the case of an abstract class, a class may extend only one abstract class | A Class may implement several interfaces |
An abstract class can have non-abstract methods | All methods of an Interface are abstract |
An abstract class can have instance variables | An Interface cannot have instance variables |
An abstract class can have any visibility: public, private, protected | An Interface visibility must be public (or) none |
If we add a new method to an abstract class then we have the option of providing default implementation and therefore all the existing code might work properly | If we add a new method to an Interface then we have to track down all the implementations of the interface and define implementation for the new method |
An abstract class can contain constructors | An Interface cannot contain constructors |
Abstract classes are fast | Interfaces are slow as it requires extra indirection to find the corresponding method in the actual class |
Q6. What is inheritance in Java?
Inheritance in Java is the concept where the properties of one class can be inherited by the other. It helps to reuse the code and establish a relationship between different classes. Inheritance is performed between two types of classes:
- Parent class (Super or Base class)
- Child class (Subclass or Derived class)
A class which inherits the properties is known as Child Class whereas a class whose properties are inherited is known as Parent class.
Q7. What are the different types of inheritance in Java?
Java supports four types of inheritance which are:
- Single Inheritance: In single inheritance, one class inherits the properties of another i.e there will be only one parent as well as one child class.
- Multilevel Inheritance: When a class is derived from a class which is also derived from another class, i.e. a class having more than one parent class but at different levels, such type of inheritance is called Multilevel Inheritance.
- Hierarchical Inheritance: When a class has more than one child classes (subclasses) or in other words, more than one child classes have the same parent class, then such kind of inheritance is known as hierarchical.
- Hybrid Inheritance: Hybrid inheritance is a combination of two or more types of inheritance.
Q8. What is method overloading and method overriding?
Method Overloading :
- In Method Overloading, Methods of the same class shares the same name but each method must have a different number of parameters or parameters having different types and order.
- Method Overloading is to “add” or “extend” more to the method’s behavior.
- It is a compile-time polymorphism.
- The methods must have a different signature.
- It may or may not need inheritance in Method Overloading.
Let’s take a look at the example below to understand it better.
class Adder {
Static int add(int a, int b)
{
return a+b;
}
Static double add( double a, double b)
{
return a+b;
}
public static void main(String args[])
{
System.out.println(Adder.add(11,11));
System.out.println(Adder.add(12.3,12.6));
}}
Method Overriding:
- In Method Overriding, the subclass has the same method with the same name and exactly the same number and type of parameters and same return type as a superclass.
- Method Overriding is to “Change” existing behavior of the method.
- It is a run time polymorphism.
- The methods must have the same signature.
- It always requires inheritance in Method Overriding.
Let’s take a look at the example below to understand it better.
class Car {
void run(){
System.out.println(“car is running”);
}
Class Audi extends Car{
void run()
{
System.out.prinltn("Audi is running safely with 100km");
}
public static void main( String args[])
{
Car b=new Audi();
b.run();
}
}
Q9. Can you override a private or static method in Java?
You cannot override a private or static method in Java. If you create a similar method with the same return type and same method arguments in child class then it will hide the superclass method; this is known as method hiding. Similarly, you cannot override a private method in subclass because it’s not accessible there. What you can do is create another private method with the same name in the child class. Let’s take a look at the example below to understand it better.
class Base {
private static void display() {
System.out.println("Static or class method from Base");
}
public void print() {
System.out.println("Non-static or instance method from Base");
}
class Derived extends Base {
private static void display() {
System.out.println("Static or class method from Derived");
}
public void print() {
System.out.println("Non-static or instance method from Derived");
}
public class test {
public static void main(String args[])
{
Base obj= new Derived();
obj1.display();
obj1.print();
}
}
Q10. What is multiple inheritance? Is it supported by Java?
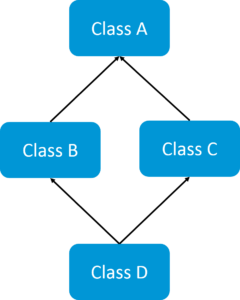
If a child class inherits the property from multiple classes is known as multiple inheritance. Java does not allow to extend multiple classes.
The problem with multiple inheritance is that if multiple parent classes have the same method name, then at runtime it becomes difficult for the compiler to decide which method to execute from the child class.
Therefore, Java doesn’t support multiple inheritance. The problem is commonly referred to as Diamond Problem.
In case you are facing any challenges with these java interview questions, please comment on your problems in the section below.
Q11. What is encapsulation in Java?
Encapsulation is a mechanism where you bind your data(variables) and code(methods) together as a single unit. Here, the data is hidden from the outer world and can be accessed only via current class methods. This helps in protecting the data from any unnecessary modification. We can achieve encapsulation in Java by:
- Declaring the variables of a class as private.
- Providing public setter and getter methods to modify and view the values of the variables.
Q12. What is an association?
Association is a relationship where all object have their own lifecycle and there is no owner. Let’s take the example of Teacher and Student. Multiple students can associate with a single teacher and a single student can associate with multiple teachers but there is no ownership between the objects and both have their own lifecycle. These relationships can be one to one, one to many, many to one and many to many.
Q13. What do you mean by aggregation?
An aggregation is a specialized form of Association where all object has their own lifecycle but there is ownership and child object can not belong to another parent object. Let’s take an example of Department and teacher. A single teacher can not belong to multiple departments, but if we delete the department teacher object will not destroy.
Q14. What is composition in Java?
Composition is again a specialized form of Aggregation and we can call this as a “death” relationship. It is a strong type of Aggregation. Child object does not have their lifecycle and if parent object deletes all child object will also be deleted. Let’s take again an example of a relationship between House and rooms. House can contain multiple rooms there is no independent life of room and any room can not belongs to two different houses if we delete the house room will automatically delete.
Q15. What is a marker interface?
A Marker interface can be defined as the interface having no data member and member functions. In simpler terms, an empty interface is called the Marker interface. The most common examples of Marker interface in Java are Serializable, Cloneable etc. The marker interface can be declared as follows.
public interface Serializable{}
Q16. What is object cloning in Java?
Object cloning in Java is the process of creating an exact copy of an object. It basically means the ability to create an object with a similar state as the original object. To achieve this, Java provides a method clone() to make use of this functionality. This method creates a new instance of the class of the current object and then initializes all its fields with the exact same contents of corresponding fields. To object clone(), the marker interface java.lang.Cloneable must be implemented to avoid any runtime exceptions. One thing you must note is Object clone() is a protected method, thus you need to override it.
Q17. What is a copy constructor in Java?
Copy constructor is a member function that is used to initialize an object using another object of the same class. Though there is no need for copy constructor in Java since all objects are passed by reference. Moreover, Java does not even support automatic pass-by-value.
Q18. What is a constructor overloading in Java?
In Java, constructor overloading is a technique of adding any number of constructors to a class each having a different parameter list. The compiler uses the number of parameters and their types in the list to differentiate the overloaded constructors.
class Demo
{
int i;
public Demo(int a)
{
i=k;
}
public Demo(int a, int b)
{
//body
}
}
In case you are facing any challenges with these java interview questions, please comment on your problems in the section below. Apart from this Java Interview Questions Blog, if you want to get trained from professionals on this technology, you can opt for a structured training from edureka!
Servlets – Java Interview Questions
Q1. What is a servlet?
- Java Servlet is server-side technologies to extend the capability of web servers by providing support for dynamic response and data persistence.
- The javax.servlet and javax.servlet.http packages provide interfaces and classes for writing our own servlets.
- All servlets must implement the javax.servlet.Servlet interface, which defines servlet lifecycle methods. When implementing a generic service, we can extend the GenericServlet class provided with the Java Servlet API. The HttpServlet class provides methods, such as doGet() and doPost(), for handling HTTP-specific services.
- Most of the times, web applications are accessed using HTTP protocol and thats why we mostly extend HttpServlet class. Servlet API hierarchy is shown in below image.
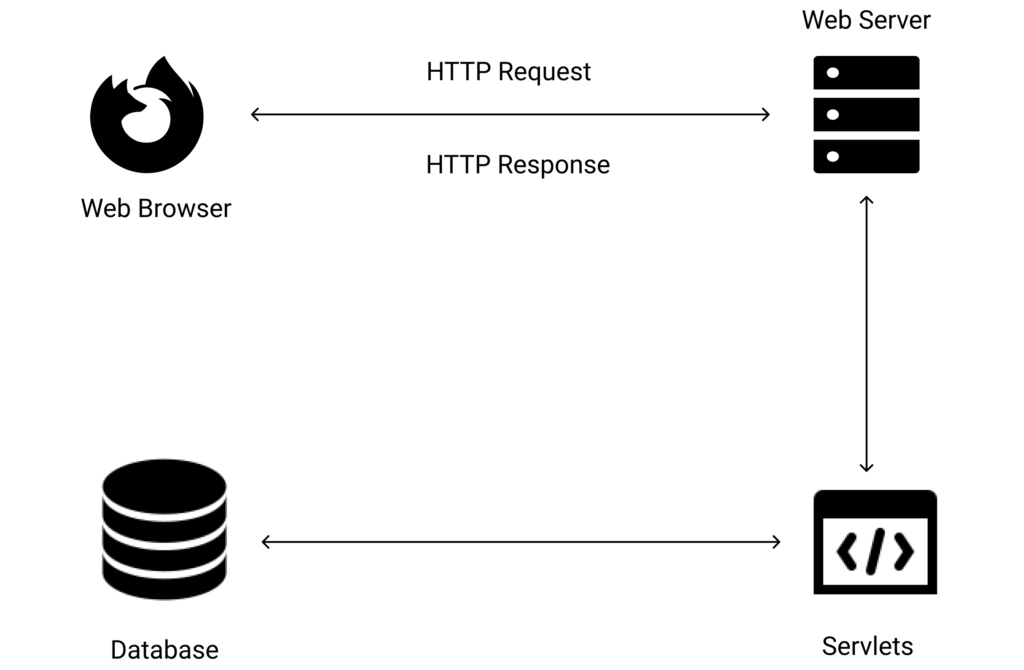
Q2. What are the differences between Get and Post methods?
Get | Post |
---|---|
Limited amount of data can be sent because data is sent in header. | Large amount of data can be sent because data is sent in body. |
Not Secured because data is exposed in URL bar. | Secured because data is not exposed in URL bar. |
Can be bookmarked | Cannot be bookmarked |
Idempotent | Non-Idempotent |
It is more efficient and used than Post | It is less efficient and used |
Q3. What is Request Dispatcher?
RequestDispatcher interface is used to forward the request to another resource that can be HTML, JSP or another servlet in same application. We can also use this to include the content of another resource to the response.
There are two methods defined in this interface:
1.void forward()
2.void include()
Q4. What are the differences between forward() method and sendRedirect() methods?
forward() method | SendRedirect() method |
---|---|
forward() sends the same request to another resource. | sendRedirect() method sends new request always because it uses the URL bar of the browser. |
forward() method works at server side. | sendRedirect() method works at client side. |
forward() method works within the server only. | sendRedirect() method works within and outside the server. |
Q5. What is the life-cycle of a servlet?
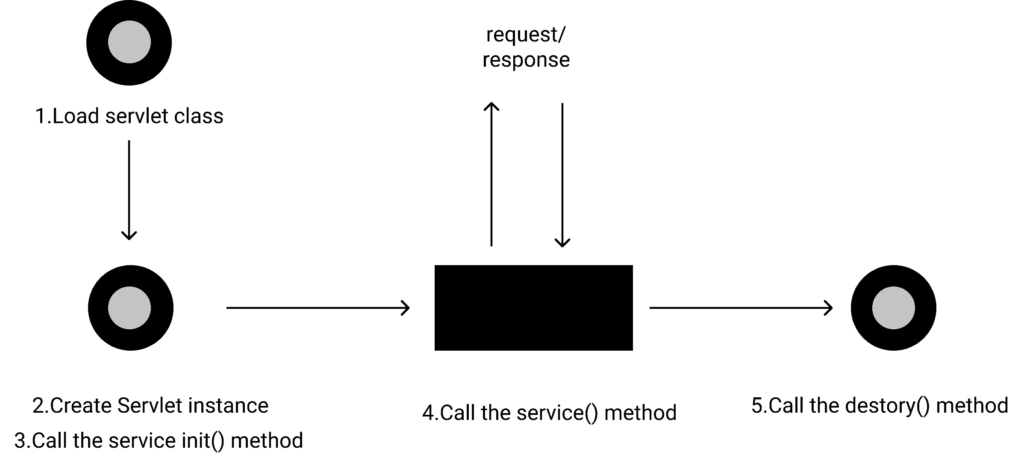
There are 5 stages in the lifecycle of a servlet:
- Servlet is loaded
- Servlet is instantiated
- Servlet is initialized
- Service the request
- Servlet is destroyed
Q6. How does cookies work in Servlets?
- Cookies are text data sent by server to the client and it gets saved at the client local machine.
- Servlet API provides cookies support through javax.servlet.http.Cookie class that implements Serializable and Cloneable interfaces.
- HttpServletRequest getCookies() method is provided to get the array of Cookies from request, since there is no point of adding Cookie to request, there are no methods to set or add cookie to request.
- Similarly HttpServletResponse addCookie(Cookie c) method is provided to attach cookie in response header, there are no getter methods for cookie.
Q7. What are the differences between ServletContext vs ServletConfig?
The difference between ServletContext and ServletConfig in Servlets JSP is in below tabular format.
ServletConfig | ServletContext |
---|---|
Servlet config object represent single servlet | It represent whole web application running on particular JVM and common for all the servlet |
Its like local parameter associated with particular servlet | Its like global parameter associated with whole application |
It’s a name value pair defined inside the servlet section of web.xml file so it has servlet wide scope | ServletContext has application wide scope so define outside of servlet tag in web.xml file. |
getServletConfig() method is used to get the config object | getServletContext() method is used to get the context object. |
for example shopping cart of a user is a specific to particular user so here we can use servlet config | To get the MIME type of a file or application session related information is stored using servlet context object. |
Q8. What are the different methods of session management in servlets?
Session is a conversational state between client and server and it can consists of multiple request and response between client and server. Since HTTP and Web Server both are stateless, the only way to maintain a session is when some unique information about the session (session id) is passed between server and client in every request and response.
Some of the common ways of session management in servlets are:
- User Authentication
- HTML Hidden Field
- Cookies
- URL Rewriting
- Session Management API
JDBC – Java Interview Questions
1. What is JDBC Driver?
JDBC Driver is a software component that enables java application to interact with the database. There are 4 types of JDBC drivers:
- JDBC-ODBC bridge driver
- Native-API driver (partially java driver)
- Network Protocol driver (fully java driver)
- Thin driver (fully java driver)
2. What are the steps to connect to a database in java?
- Registering the driver class
- Creating connection
- Creating statement
- Executing queries
- Closing connection
3. What are the JDBC API components?
The java.sql package contains interfaces and classes for JDBC API.
Interfaces:
- Connection
- Statement
- PreparedStatement
- ResultSet
- ResultSetMetaData
- DatabaseMetaData
- CallableStatement etc.
Classes:
- DriverManager
- Blob
- Clob
- Types
- SQLException etc.
4. What is the role of JDBC DriverManager class?
The DriverManager class manages the registered drivers. It can be used to register and unregister drivers. It provides factory method that returns the instance of Connection.
5. What is JDBC Connection interface?
The Connection interface maintains a session with the database. It can be used for transaction management. It provides factory methods that returns the instance of Statement, PreparedStatement, CallableStatement and DatabaseMetaData.
6. What is the purpose of JDBC ResultSet interface?
The ResultSet object represents a row of a table. It can be used to change the cursor pointer and get the information from the database.
7. What is JDBC ResultSetMetaData interface?
The ResultSetMetaData interface returns the information of table such as total number of columns, column name, column type etc.
8. What is JDBC DatabaseMetaData interface?
The DatabaseMetaData interface returns the information of the database such as username, driver name, driver version, number of tables, number of views etc.
9. What do you mean by batch processing in JDBC?
Batch processing helps you to group related SQL statements into a batch and execute them instead of executing a single query. By using batch processing technique in JDBC, you can execute multiple queries which makes the performance faster.
10. What is the difference between execute, executeQuery, executeUpdate?
Statement execute(String query) is used to execute any SQL query and it returns TRUE if the result is an ResultSet such as running Select queries. The output is FALSE when there is no ResultSet object such as running Insert or Update queries. We can use getResultSet() to get the ResultSet and getUpdateCount() method to retrieve the update count.
Statement executeQuery(String query) is used to execute Select queries and returns the ResultSet. ResultSet returned is never null even if there are no records matching the query. When executing select queries we should use executeQuery method so that if someone tries to execute insert/update statement it will throw java.sql.SQLException with message “executeQuery method can not be used for update”.
Statement executeUpdate(String query) is used to execute Insert/Update/Delete (DML) statements or DDL statements that returns nothing. The output is int and equals to the row count for SQL Data Manipulation Language (DML) statements. For DDL statements, the output is 0.
You should use execute() method only when you are not sure about the type of statement else use executeQuery or executeUpdate method.
Q11. What do you understand by JDBC Statements?
JDBC statements are basically the statements which are used to send SQL commands to the database and retrieve data back from the database. Various methods like execute(), executeUpdate(), executeQuery, etc. are provided by JDBC to interact with the database.
JDBC supports 3 types of statements:
- Statement: Used for general purpose access to the database and executes a static SQL query at runtime.
- PreparedStatement: Used to provide input parameters to the query during execution.
- CallableStatement: Used to access the database stored procedures and helps in accepting runtime parameters.
In case you are facing any challenges with these java interview questions, please comment your problems in the section below. Apart from this Java Interview Questions Blog, if you want to get trained from professionals on this technology, you can opt for a structured training from edureka!
JSP – Java Questions
1. What are the life-cycle methods for a jsp?
Methods | Description |
public void jspInit() | It is invoked only once, same as init method of servlet. |
public void _jspService(ServletRequest request,ServletResponse)throws ServletException,IOException | It is invoked at each request, same as service() method of servlet. |
public void jspDestroy() | It is invoked only once, same as destroy() method of servlet. |
2. What are the JSP implicit objects?
JSP provides 9 implicit objects by default. They are as follows:
Object | Type |
1) out | JspWriter |
2) request | HttpServletRequest |
3) response | HttpServletResponse |
4) config | ServletConfig |
5) session | HttpSession |
6) application | ServletContext |
7) pageContext | PageContext |
8) page | Object |
9) exception | Throwable |
3. What are the differences between include directive and include action?
include directive | include action |
The include directive includes the content at page translation time. | The include action includes the content at request time. |
The include directive includes the original content of the page so page size increases at runtime. | The include action doesn’t include the original content rather invokes the include() method of Vendor provided class. |
It’s better for static pages. | It’s better for dynamic pages. |
4. How to disable caching on back button of the browser?
<%
response.setHeader(“Cache-Control”,”no-store”);
response.setHeader(“Pragma”,”no-cache”);
response.setHeader (“Expires”, “0”); //prevents caching at the proxy server
%>
5. What are the different tags provided in JSTL?
There are 5 type of JSTL tags.
- core tags
- sql tags
- xml tags
- internationalization tags
- functions tags
6. How to disable session in JSP?
<%@ page session=“false” %>
7. How to delete a Cookie in a JSP?
The following code explains how to delete a Cookie in a JSP :
Cookie mycook = new Cookie("name1","value1");
response.addCookie(mycook1);
Cookie killmycook = new Cookie("mycook1","value1");
killmycook . set MaxAge ( 0 );
killmycook . set Path ("/");
killmycook . addCookie ( killmycook 1 );
8. Explain the jspDestroy() method.
jspDestry() method is invoked from javax.servlet.jsp.JspPage interface whenever a JSP page is about to be destroyed. Servlets destroy methods can be easily overridden to perform cleanup, like when closing a database connection.
9. How is JSP better than Servlet technology?
JSP is a technology on the server’s side to make content generation simple. They are document-centric, whereas servlets are programs. A Java server page can contain fragments of Java program, which execute and instantiate Java classes. However, they occur inside an HTML template file. It provides the framework for the development of a Web Application.
10. Why should we not configure JSP standard tags in web.xml?
We don’t need to configure JSP standard tags in web.xml because when container loads the web application and find TLD files, it automatically configures them to be used directly in the application JSP pages. We just need to include it in the JSP page using taglib directive.
11. How will you use JSP EL in order to get the HTTP method name?
Using pageContext JSP EL implicit object you can get the request object reference and make use of the dot operator to retrieve the HTTP method name in the JSP page. The JSP EL code for this purpose will look like ${pageContext.request.method}.
Exception and Thread Java Interview Questions
Q1. What is the difference between Error and Exception?
An error is an irrecoverable condition occurring at runtime. Such as OutOfMemory error. These JVM errors you cannot repair them at runtime. Though error can be caught in the catch block but the execution of application will come to a halt and is not recoverable.
While exceptions are conditions that occur because of bad input or human error etc. e.g. FileNotFoundException will be thrown if the specified file does not exist. Or a NullPointerException will take place if you try using a null reference. In most of the cases it is possible to recover from an exception (probably by giving the user feedback for entering proper values etc.
Q2. How can you handle Java exceptions?
There are five keywords used to handle exceptions in Java:
- try
- catch
- finally
- throw
- throws
Q3. What are the differences between Checked Exception and Unchecked Exception?
Checked Exception
- The classes that extend Throwable class except RuntimeException and Error are known as checked exceptions.
- Checked exceptions are checked at compile-time.
- Example: IOException, SQLException etc.
Unchecked Exception
- The classes that extend RuntimeException are known as unchecked exceptions.
- Unchecked exceptions are not checked at compile-time.
- Example: ArithmeticException, NullPointerException etc.
Q4. What purpose do the keywords final, finally, and finalize fulfill?
Final:
Final is used to apply restrictions on class, method, and variable. A final class can’t be inherited, final method can’t be overridden and final variable value can’t be changed. Let’s take a look at the example below to understand it better.
class FinalVarExample {
public static void main( String args[])
{
final int a=10; // Final variable
a=50; //Error as value can't be changed
}
Finally
Finally is used to place important code, it will be executed whether the exception is handled or not. Let’s take a look at the example below to understand it better.
class FinallyExample {
public static void main(String args[]){
try {
int x=100;
}
catch(Exception e) {
System.out.println(e);
}
finally {
System.out.println("finally block is executing");}
}}
}
Finalize
Finalize is used to perform clean up processing just before the object is garbage collected. Let’s take a look at the example below to understand it better.
class FinalizeExample {
public void finalize() {
System.out.println("Finalize is called");
}
public static void main(String args[])
{
FinalizeExample f1=new FinalizeExample();
FinalizeExample f2=new FinalizeExample();
f1= NULL;
f2=NULL;
System.gc();
}
}
Q5. What are the differences between throw and throws?
throw keyword | throws keyword |
Throw is used to explicitly throw an exception. | Throws is used to declare an exception. |
Checked exceptions can not be propagated with throw only. | Checked exception can be propagated with throws. |
Throw is followed by an instance. | Throws is followed by class. |
Throw is used within the method. | Throws is used with the method signature. |
You cannot throw multiple exception | You can declare multiple exception e.g. public void method()throws IOException,SQLException. |
Q6. What is exception hierarchy in java?
The hierarchy is as follows:
- Throwable is a parent class of all Exception classes.
- There are two types of Exceptions: Checked exceptions and UncheckedExceptions or RunTimeExceptions.
- Both types of exceptions extends Exception class whereas errors are further classified into Virtual Machine error and Assertion error.
Q7. How to create a custom Exception?
To create you own exception extend the Exception class or any of its subclasses.
- class New1Exception extends Exception { } // this will create Checked Exception
- class NewException extends IOException { } // this will create Checked exception
- class NewException extends NullPonterExcpetion { } // this will create UnChecked exception
Q8. What are the important methods of Java Exception Class?
Exception and all of it’s subclasses doesn’t provide any specific methods and all of the methods are defined in the base class Throwable.
- String getMessage() – This method returns the message String of Throwable and the message can be provided while creating the exception through it’s constructor.
- String getLocalizedMessage() – This method is provided so that subclasses can override it to provide locale specific message to the calling program. Throwable class implementation of this method simply use getMessage() method to return the exception message.
- Synchronized Throwable getCause() – This method returns the cause of the exception or null id the cause is unknown.
- String toString() – This method returns the information about Throwable in String format, the returned String contains the name of Throwable class and localized message.
- void printStackTrace() – This method prints the stack trace information to the standard error stream, this method is overloaded and we can pass PrintStream or PrintWriter as an argument to write the stack trace information to the file or stream.
Q9. What are the differences between processes and threads?
Process | Thread | |
Definition | An executing instance of a program is called a process. | A thread is a subset of the process. |
Communication | Processes must use inter-process communication to communicate with sibling processes. | Threads can directly communicate with other threads of its process. |
Control | Processes can only exercise control over child processes. | Threads can exercise considerable control over threads of the same process. |
Changes | Any change in the parent process does not affect child processes. | Any change in the main thread may affect the behavior of the other threads of the process. |
Memory | Run in separate memory spaces. | Run in shared memory spaces. |
Controlled by | Process is controlled by the operating system. | Threads are controlled by programmer in a program. |
Dependence | Processes are independent. | Threads are dependent. |
Q10. What is a finally block? Is there a case when finally will not execute?
Finally block is a block which always executes a set of statements. It is always associated with a try block regardless of any exception that occurs or not.
Yes, finally will not be executed if the program exits either by calling System.exit() or by causing a fatal error that causes the process to abort.
Q11. What is synchronization?
Synchronization refers to multi-threading. A synchronized block of code can be executed by only one thread at a time. As Java supports execution of multiple threads, two or more threads may access the same fields or objects. Synchronization is a process which keeps all concurrent threads in execution to be in sync. Synchronization avoids memory consistency errors caused due to inconsistent view of shared memory. When a method is declared as synchronized the thread holds the monitor for that method’s object. If another thread is executing the synchronized method the thread is blocked until that thread releases the monitor.
Q12. Can we write multiple catch blocks under single try block?
Yes we can have multiple catch blocks under single try block but the approach should be from specific to general. Let’s understand this with a programmatic example.
public class Example {
public static void main(String args[]) {
try {
int a[]= new int[10];
a[10]= 10/0;
}
catch(ArithmeticException e)
{
System.out.println("Arithmetic exception in first catch block");
}
catch(ArrayIndexOutOfBoundsException e)
{
System.out.println("Array index out of bounds in second catch block");
}
catch(Exception e)
{
System.out.println("Any exception in third catch block");
}
}
Q13. What are the important methods of Java Exception Class?
Methods are defined in the base class Throwable. Some of the important methods of Java exception class are stated below.
- String getMessage() – This method returns the message String about the exception. The message can be provided through its constructor.
- public StackTraceElement[] getStackTrace() – This method returns an array containing each element on the stack trace. The element at index 0 represents the top of the call stack whereas the last element in the array represents the method at the bottom of the call stack.
- Synchronized Throwable getCause() – This method returns the cause of the exception or null id as represented by a Throwable object.
- String toString() – This method returns the information in String format. The returned String contains the name of Throwable class and localized message.
- void printStackTrace() – This method prints the stack trace information to the standard error stream.
Q14. What is OutOfMemoryError in Java?
OutOfMemoryError is the subclass of java.lang.Error which generally occurs when our JVM runs out of memory.
Q15. What is a Thread?
A thread is the smallest piece of programmed instructions which can be executed independently by a scheduler. In Java, all the programs will have at least one thread which is known as the main thread. This main thread is created by the JVM when the program starts its execution. The main thread is used to invoke the main() of the program.
Q16. What are the two ways to create a thread?
In Java, threads can be created in the following two ways:-
- By implementing the Runnable interface.
- By extending the Thread
Q17. What are the different types of garbage collectors in Java?
Garbage collection in Java a program which helps in implicit memory management. Since in Java, using the new keyword you can create objects dynamically, which once created will consume some memory. Once the job is done and there are no more references left to the object, Java using garbage collection destroys the object and relieves the memory occupied by it. Java provides four types of garbage collectors:
- Serial Garbage Collector
- Parallel Garbage Collector
- CMS Garbage Collector
- G1 Garbage Collector
Hope this article on Java Interview Questions is helpful to you .Feel free comments for suggestion to improve and ask a question !